How to Break a For Loop: A Comprehensive Guide

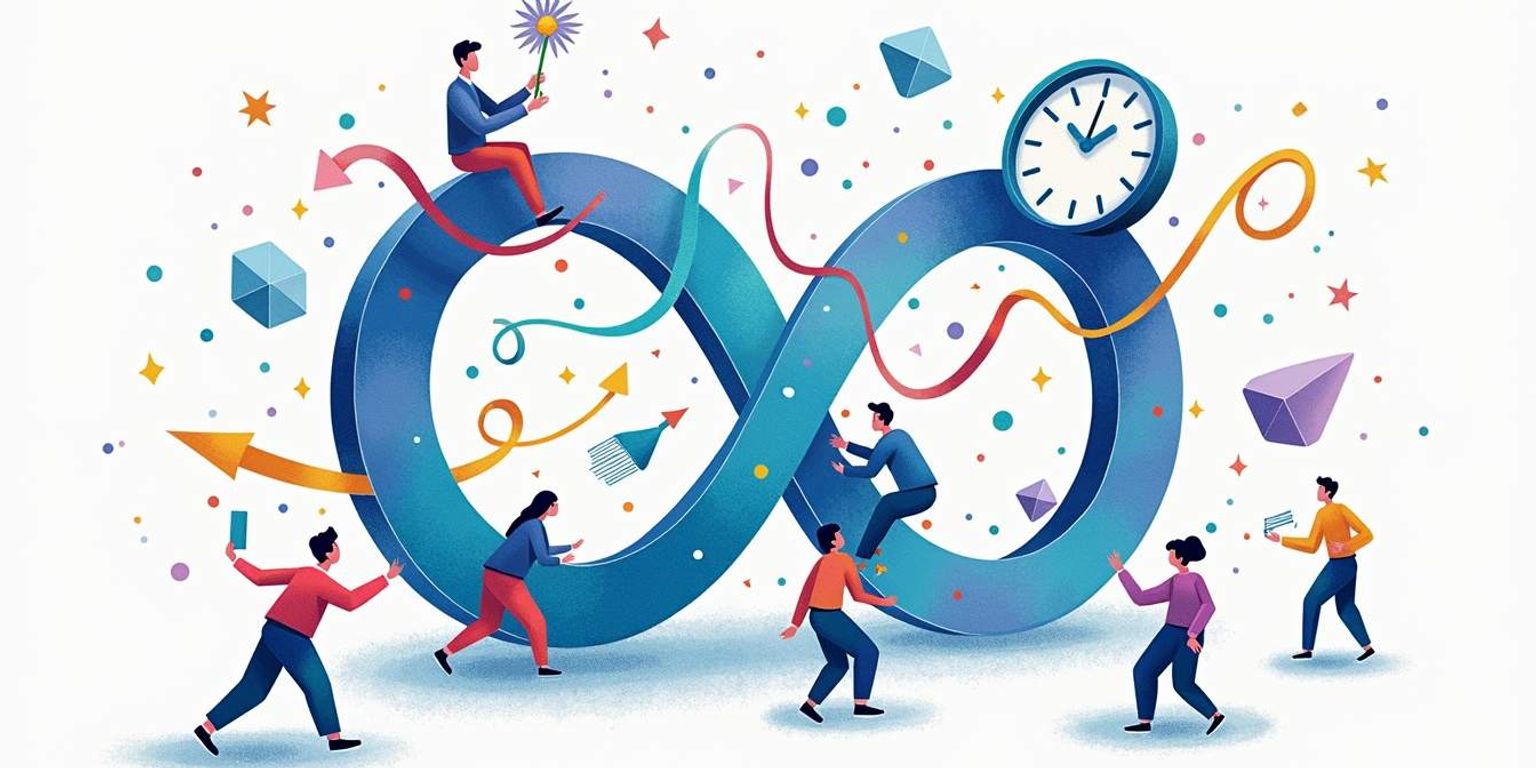
How to Break a For Loop: A Comprehensive Guide
In programming, loops are essential for executing repetitive tasks efficiently. Among the various types of loops, the for
loop is one of the most commonly used structures. However, there are times when it becomes necessary to exit a loop prematurely. This article delves into the intricacies of breaking a for
loop, providing a comprehensive guide to understanding and implementing this functionality effectively.
Understanding the For Loop
The for
loop is a control flow statement that allows code to be executed repeatedly based on a given condition. It consists of three main parts: initialization, condition, and increment/decrement. This structure makes it particularly useful for iterating over arrays or collections, enabling developers to efficiently process data in a structured manner.
Basic Syntax
The syntax of a for
loop is straightforward. It typically looks like this:
for (initialization; condition; increment) { // Code to be executed}
In this structure, the initialization sets up the loop variable, the condition determines how long the loop will run, and the increment updates the loop variable after each iteration. This clarity in syntax allows programmers to quickly grasp the flow of the loop, making it easier to debug and maintain code.
Common Use Cases
For loops are widely used in various programming scenarios. They are particularly effective for:
- Iterating through arrays or lists
- Performing repetitive calculations
- Generating sequences of numbers
Understanding these use cases can help programmers utilize for
loops more effectively in their code. For instance, when dealing with large datasets, a for
loop can streamline the process of searching for specific elements or applying transformations to each item. Additionally, they are invaluable in scenarios where the number of iterations is known beforehand, such as when processing user input or managing game mechanics.
Moreover, the versatility of the for
loop extends beyond simple iterations. It can be nested within other loops, allowing for complex operations such as matrix manipulation or multi-dimensional data processing. This nesting capability opens up a world of possibilities for tackling intricate programming challenges, making the for
loop an essential tool in a developer's toolkit.
Why Break a For Loop?
While for
loops are powerful, there are situations where continuing to execute the loop becomes unnecessary or inefficient. Breaking a loop allows programmers to exit early based on specific conditions, enhancing performance and improving code readability.
Performance Optimization
In large datasets, continuing to iterate through every element may lead to performance bottlenecks. By breaking the loop when a certain condition is met, developers can optimize their code, saving both time and computational resources. For example, in scenarios where data is being processed from a database, if a specific threshold is reached, it may be more efficient to halt further processing rather than continuing through potentially millions of records. This not only speeds up the execution but also reduces the load on the system, allowing it to allocate resources to other tasks.
Conditional Logic
Sometimes, the logic of the program dictates that a loop should terminate based on user input or other dynamic conditions. For instance, if a user is searching for a specific item in a list, breaking the loop upon finding that item can lead to a more responsive application. Additionally, this technique can be particularly useful in interactive applications where user experience is paramount. By implementing a break statement, developers can ensure that the application responds swiftly to user actions, thereby enhancing overall satisfaction. Furthermore, this approach can also be applied in scenarios such as game development, where a loop might be used to check for collision detection; breaking the loop as soon as a collision is detected can significantly improve the game's performance and responsiveness.
How to Break a For Loop
Breaking a for
loop is typically done using the break
statement. This command immediately exits the loop, regardless of the loop's condition. Here’s how it works:
Using the Break Statement
The break
statement can be placed within the loop's body. When the program execution reaches this statement, it will terminate the loop and continue executing the code that follows it. Here’s a simple example:
for (int i = 0; i < 10; i++) { if (i == 5) { break; // Exit the loop when i equals 5 } System.out.println(i);}
In this example, the loop will print numbers 0 through 4, and then exit when i
equals 5. This behavior is particularly useful when you want to stop processing once a certain condition is met, which can help optimize performance by avoiding unnecessary iterations.
Nested Loops and Breaking
When dealing with nested loops, the break
statement will only exit the innermost loop. This is an important consideration when designing algorithms that require multiple levels of iteration. To break out of outer loops, a labeled break can be used in some programming languages, such as Java:
outerLoop:for (int i = 0; i < 5; i++) { for (int j = 0; j < 5; j++) { if (j == 3) { break outerLoop; // Exits both loops } System.out.println("i: " + i + ", j: " + j); }}
This example demonstrates how to exit both loops when a certain condition is met. Understanding how to effectively use labeled breaks can greatly enhance control over complex iterations, allowing for more readable and maintainable code.
Moreover, breaking out of loops can also be combined with other control flow statements, such as continue
, which skips the current iteration and proceeds to the next one. This combination can be particularly powerful when filtering data or managing complex conditions within your loops. For instance, you might want to break out of a loop when a certain threshold is reached, while continuing to process other elements that do not meet this threshold. Such strategies can lead to more efficient algorithms, especially when working with large datasets or performance-critical applications.
Best Practices for Breaking Loops
While breaking loops can enhance performance, it is essential to use this technique judiciously. Here are some best practices to consider:
Maintain Readability
Code readability is crucial for maintaining and updating software. When using the break
statement, ensure that its purpose is clear. Adding comments can help other developers (or your future self) understand why the loop is being exited.
Avoid Overuse of Break
Using break
excessively can lead to convoluted logic that is difficult to follow. It is often better to structure the loop's condition to achieve the desired outcome without relying on breaks. This approach can make the code cleaner and easier to debug.
Testing and Debugging
Thoroughly test code that utilizes break statements to ensure that it behaves as expected. Edge cases should be considered to avoid unintended consequences, such as skipping necessary iterations or exiting loops prematurely.
Real-World Applications
Breaking loops is not just a theoretical concept; it has practical applications in various fields, including data processing, game development, and web applications.
Data Processing
In data processing tasks, such as searching through large datasets, breaking a loop can significantly improve performance. For example, when searching for a specific record in a database, the loop can terminate as soon as the record is found, reducing the time complexity of the operation.
Game Development
In game development, loops are often used for rendering frames or processing player inputs. Breaking a loop can be essential for handling events, such as pausing the game or responding to user actions. This ensures a smooth and responsive gaming experience.
Common Pitfalls to Avoid
While breaking loops can be beneficial, there are common pitfalls that programmers should be aware of to avoid introducing bugs into their code.
Infinite Loops
One of the most significant risks when using loops is the potential for creating infinite loops. This can occur if the loop's exit condition is never met. Always ensure that the logic within the loop allows for eventual termination, whether through a break statement or a condition that will eventually evaluate to false.
Logic Errors
Breaking a loop prematurely can lead to logic errors if not handled correctly. For instance, if a loop is designed to perform a series of calculations, breaking it too early might result in incomplete data processing. It is crucial to carefully consider the implications of breaking a loop in the context of the overall program logic.
Conclusion
Breaking a for
loop is a powerful technique that can enhance the efficiency and readability of code. By understanding when and how to use the break
statement, programmers can create more responsive and optimized applications. Whether in data processing, game development, or web applications, the ability to exit loops when necessary is a valuable skill.
As the programming landscape evolves, tools and frameworks continue to emerge that simplify these processes. For instance, Clarify is developing a next-generation CRM that incorporates advanced features to streamline data management and enhance user experience. By leveraging such tools, developers can focus on writing efficient code while the underlying systems handle the complexities of data processing.
In summary, mastering the art of breaking loops is essential for any programmer looking to improve their coding skills and deliver high-quality software solutions. With practice and careful consideration, breaking loops can become a natural part of the coding process.
Take Your Coding to the Next Level with Clarify
Just as breaking a for
loop can optimize your code, Clarify optimizes your customer relationship management. Embrace the future of CRM and harness the power of AI to unify your data, automate processes, and gain valuable insights. Don't let manual tasks slow you down—let Clarify streamline your workflow and help you focus on growing your business. Ready to experience a CRM that's designed with your needs in mind? Request access to Clarify today and transform the way you manage customer relationships.
Get our newsletter
Subscribe for weekly essays on GTM, RevTech, and Clarify’s latest updates.
Thanks for subscribing! We'll send only our best stuff. Your information will not be shared and you can unsubscribe at any time.